Our New Relic React Native agent monitors your React Native mobile app and provides deep insights into your app's performance, errors, and user experience. Written in JavaScript, the React Native agent includes all New Relic mobile monitoring features we offer for native mobile apps. Once you install and configure the React Native agent, you'll be able to:
- Capture JavaScript errors Identify and fix problems quickly.
- Track network requests: See how your app interacts with the backend.
- Use distributed tracing: Drill down into handled exceptions and find the root cause.
- Create custom events and metrics: Understand how your users interact with your app.
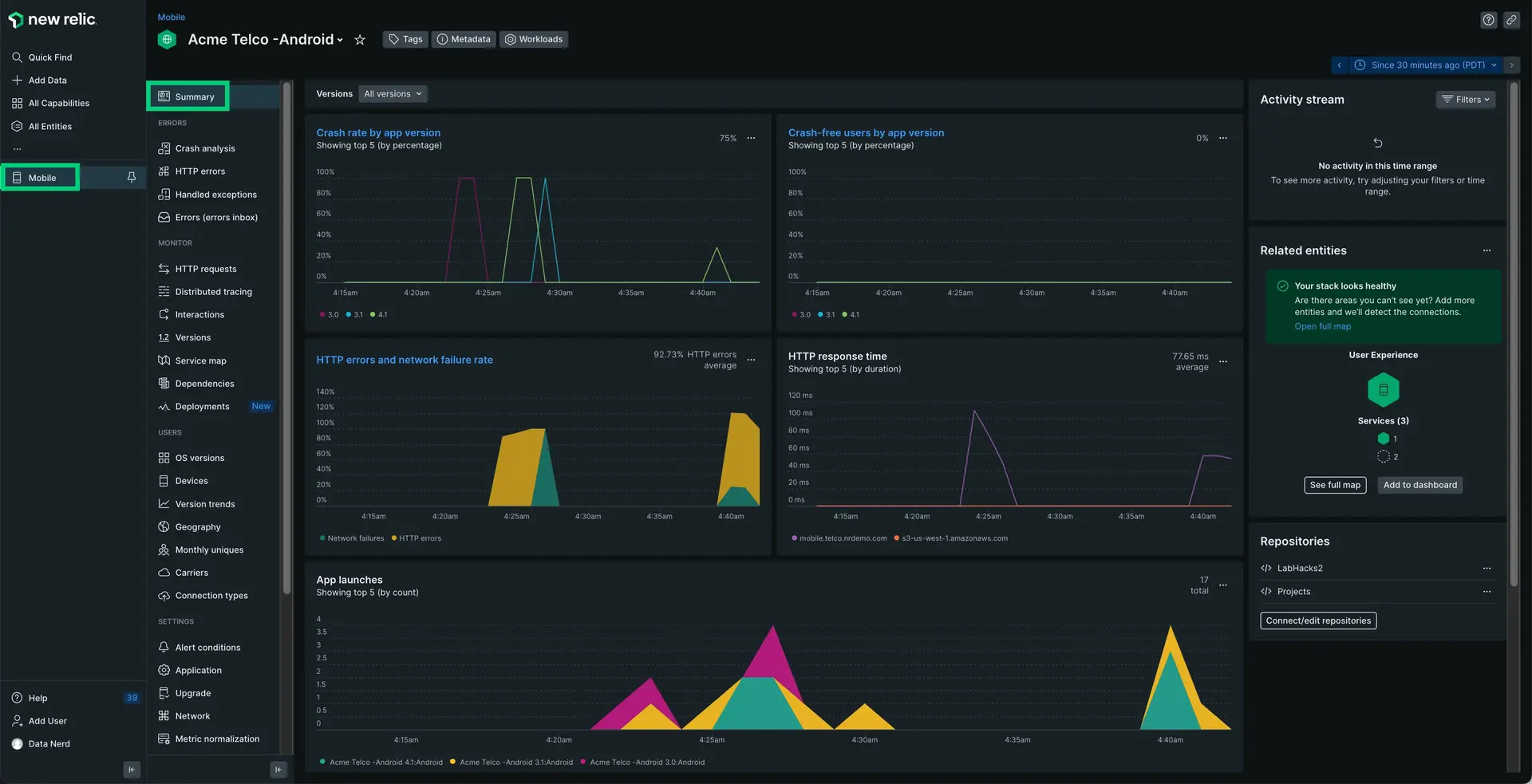
one.newrelic.com > All capabilities > Mobile > (select an app) > Summary: The React Native agent sends data to New Relic, where you can analyze crash data, network traffic, and other info on your hybrid app.
The agent allows your team to understand the health of your hybrid app regardless of the platform. We empower your team to make more informed development choices by providing insight into Javascript errors, distributed tracing, network instrumentation, and more.
Compatibility and requirements
Before you install the React Native agent, ensure your app meets these requirements:
Native support levels are based on React Native requirements.
(Recommended) Guided installation
To install the React Native agent, follow our guided install, located directly in the UI.
Manual installation
If you need to install the agent manually, follow these steps:
Add the React Native agent
Run the following:
$npm i newrelic-react-native-agent
Configure your application
Open your index.js
and add the following code to launch New Relic:
import NewRelic from "newrelic-react-native-agent";import { name, version } from "./package.json";import { Platform } from "react-native";
let appToken;if (Platform.OS === "ios") { appToken = "IOS-APP-TOKEN";} else { appToken = "ANDROID-APP-TOKEN";}
const agentConfiguration = { // Android Specific // Optional:Enable or disable collection of event data. analyticsEventEnabled: true,
// Optional:Enable or disable crash reporting. crashReportingEnabled: true,
// Optional:Enable or disable interaction tracing. Trace instrumentation still occurs, but no traces are harvested. // This will disable default and custom interactions. interactionTracingEnabled: true,
// Optional:Enable or disable reporting successful HTTP requests to the MobileRequest event type. networkRequestEnabled: true,
// Optional:Enable or disable reporting network and HTTP request errors to the MobileRequestError event type. networkErrorRequestEnabled: true, // Optional:Enable or disable capture of HTTP response bodies for HTTP error traces, and MobileRequestError events. httpRequestBodyCaptureEnabled: true, // Optional:Enable or disable agent logging. loggingEnabled: true, // Optional:Specifies the log level. Omit this field for the default log level. // Options include: ERROR (least verbose), WARNING, INFO, VERBOSE, AUDIT (most verbose). logLevel: NewRelic.LogLevel.INFO, // iOS Specific // Optional:Enable/Disable automatic instrumentation of WebViews webViewInstrumentation: true, // Optional:Set a specific collector address for sending data. Omit this field for default address. collectorAddress: "", // Optional:Set a specific crash collector address for sending crashes. Omit this field for default address. crashCollectorAddress: "", // Optional:Enable or disable reporting data using different endpoints for US government clients fedRampEnabled: false, // Optional: Enable or disable offline data storage when no internet connection is available. offlineStorageEnabled: true,
// iOS Specific // Optional: Enable or disable Background Reporting. backgroundReportingEnabled: false,
// iOS Specific // Optional: Enable or disable to use our new, more stable event system for iOS agent. newEventSystemEnabled: false,};
NewRelic.startAgent(appToken, agentConfiguration);NewRelic.setJSAppVersion(version);AppRegistry.registerComponent(name, () => App);
Copy/paste your app token(s)
In the code above, paste your application token(s) into appToken = ""
in the code above. If you deployed your hybrid app to both iOS and Android platforms, you'll need to add two separate tokens: one for iOS and one for Android. If you use the same app token, you won't be able to compare app performance between platforms.
To copy/paste your app token(s):
Go to one.newrelic.com > All capabilities > Mobile > (select your mobile app) > Settings.
Copy the application token.
In the code above, replace
<IOS-APP-TOKEN>
and/or<ANDROID-APP-TOKEN>
with your app token. If you're deploying to both Android and iOS, repeat this process to get the second app token.
(Android-native apps only) Install the Android agent
- Install the New Relic native Android agent.
- Add the following changes to apply the Gradle plugin:
If your project is using plugin DSL, make the following changes:
In android/app/build.gradle:
plugins { id "com.android.application" id "kotlin-android" id "com.newrelic.agent.android" version "7.5.1" //<-- include this}
Or, if your project is older, you can use the legacy newrelic
plugin ID by adding this snippet:
buildscript { ... repositories { ... mavenCentral() } dependencies { ... classpath "com.newrelic.agent.android:agent-gradle-plugin:7.5.1" }}
Apply the NewRelic plugin
to the top of the android/app/build.gradle
file:
apply plugin: "com.android.application"apply plugin: 'newrelic' // <-- include this
- Make sure your app requests
INTERNET
andACCESS_NETWORK_STATE
permissions by adding these lines to yourAndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET" /><uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
(iOS-native apps only) Install the iOS agent
To ensure that the React Native agent is compatible with all iOS frameworks, install the New Relic iOS agent:
$npx pod-install
Configure autolinking and rebuilding
The React Native New Relic library must be linked to your project and your application needs to be rebuilt. If you use React Native 0.60+, you automatically have access to autolinking, requiring no further manual installation steps.
For Android:
$npx react-native run-android
For iOS:
$cd ios/$pod install --repo-update$cd ..$npx react-native run-ios
In order to make sure that the React Native application functioning properly, you can run following commands, and fatal JS errors will show up as a crash in the New Relic UI.
For Android:
$npx react-native run-android --variant=release
For iOS:
$npx react-native run-ios --configuration Release
(Optional) Integrate with Expo
After installing the React Native agent, integration with Expo is automatic with bare workflow but requires some extra steps for custom managed workflow via config plugins.
To set up custom managed workflow, after installing our package, add the config plugin to the plugins array of your app.json
or app.config.js
.
{ "name": "my app", "plugins": ["newrelic-react-native-agent"]}
For the managed work flow, use the expo prebuild --clean
command as described in the Adding custom native code guide to rebuild your app with the plugin changes. If this command is not running, you'll get errors when starting the New Relic agent. For Expo Go users, the agent will require using native code. Since Expo Go does not support sending custom native code over-the-air, you can follow Expo's documentation on how to use custom native code in Expo Go.
(Optional) Configure instrument routing and navigation
To instrument routing and navigation for your React Native apps:
Customize the agent instrumentation
Need to customize your agent instrumentation? Our public mobile SDK API methods let you collect custom data, configure default settings, and more.
The following customizations are available for the React Native agent.
If you want to... | Use this method |
---|---|
Record breadcrumbs to track app activity that may be helpful for troubleshooting crashes. | |
Track a method as an interaction. | |
Record custom metrics. | |
Record custom errors. | |
Record custom attributes and events. | There are several ways to report custom attributes and events:
|
Track custom network requests and failures. | |
Shut down the agent. | |
Enable/disable default mobile monitoring settings. | |
Run a test crash report. |
Troubleshoot JavaScript errors
JavaScript console logs
To view JavaScript console logs in New Relic, go to one.newrelic.com > Query your data and add this query to find those JavaScript console logs:
SELECT * FROM consoleEvents SINCE 24 HOURS AGO