Syntax
Java
NewRelic.recordCustomEvent(string $eventType, [string $eventName,] map<string, object> $eventAttributes)
Kotlin
NewRelic.recordCustomEvent( eventType: String?, eventName: String?, eventAttributes: MutableMap<String?, Any?>?)
Description
Records a custom New Relic event.
Creates and records a custom event, for use in NRQL. The event includes a list of attributes, specified as a map. Unlike using setAttribute
, adding attributes to a custom event adds them only to that event; they are not session attributes.
Important considerations and best practices include:
You should limit the total number of event types to approximately five.
eventType
is meant to be used for high-level categories. For example, you might create an event typeGestures
.Do not use
eventType
to name your custom events. Create an attribute to name an event or use the optionalname
parameter. While you can create numerous custom events, it's crucial to limit the number ofeventType
s.Using the optional
name
parameter has the same effect as adding aname
key in theattributes
dictionary.name
is a keyword used for displaying your events in the New Relic UI. To create a usefulname
, you might combine several attributes (see examples).Important
As of New Relic Android agent version 5.12.0, the
recordEvent
method is deprecated and replaced withrecordCustomEvent
. TherecordEvent
method will continue to work for an unspecified period of time, but if your app containsrecordEvent
methods, New Relic recommends you replace them.Updating these methods will affect any NRQL queries and dashboards that use the deprecated event types. Be sure to adjust your NRQL queries and dashboards as needed.
Parameters
Parameter
Type
Description
$eventType
string
Required. The type of event. Do not use
$eventType
to name your custom events. Instead, use a custom attribute or the optionalname
.$eventName
dictionary
Optional. A map that includes a list of attributes that further designate subcategories to the
$eventType
. You can create attributes for any event descriptors you think will be useful. To name your custom events, create aname
attribute or use theeventName
parameter.Note: Not all object types are supported. See setAttribute for details on supported types.
Important
When setting the key for your custom attributes, be aware that there are default attributes that cannot be overridden.
Return values
Returns
true
if the event is recorded successfully, orfalse
if not.Examples
Here's an example of a basic custom event:
Java
Map attributes = new HashMap();attributes.put("attributeName1", "value1");attributes.put("attributeName1", 2);boolean eventRecorded = NewRelic.recordCustomEvent("eventType", attributes);
Kotlin
val attributes = mutableMapOf<String,Any>("attributeName1" to "value1", "attributeName1" to 2)val eventRecorded = NewRelic.recordCustomEvent("eventType", attributes)
Here's an example of a custom event with several attributes:
Java
Map attributes = new HashMap();attributes.put("make", "Ford");attributes.put("model", "ModelT");attributes.put("color", "Black");attributes.put("VIN", "123XYZ");attributes.put("maxSpeed", 12);
NewRelic.recordCustomEvent("Car", attributes);
Kotlin
val attributes = mutableMapOf<String,Any>()attributes["make"] = "Ford"attributes["model"] = "ModelT"attributes["color"] = "Black"attributes["VIN"] = "123XYZ"attributes["maxSpeed"] = 12NewRelic.recordCustomEvent("Car", attributes)
Here's an example of a custom event using the name
parameter:
Java
Map attributes = new HashMap();attributes.put("make", "Ford");attributes.put("model", "ModelT");
NewRelic.recordCustomEvent("Car", "Ford Model T", attributes);
Kotlin
val attributes = mutableMapOf<String,Any>() attributes["make"] = "Ford" attributes["model"] = "ModelT" NewRelic.recordCustomEvent("Car", "Ford Model T", attributes);
Important
As of New Relic iOS agent version 5.12.0, the recordEvent
method is deprecated and replaced with recordCustomEvent
. The recordEvent
method will continue to work for an unspecified period of time, but if your app contains recordEvent
methods, New Relic recommends you replace them.
Updating these methods will affect any queries and dashboards that use the deprecated event types. Be sure to adjust your NRQL queries and dashboards as needed.
Syntax
Objective-c
+ (BOOL) recordCustomEvent:(NSString*)eventType attributes:(NSDictionary*)attributes;
+ (BOOL) recordCustomEvent:(NSString*)eventType name:(NSString*)name attributes:(NSDictionary*)attributes;
Swift
NewRelic.recordCustomEvent(eventType: String!, attributes:[NSObject : AnyObject]!) -> Bool
NewRelic.recordCustomEvent(eventType: String!, name: String!, attributes:[NSObject : AnyObject]!) -> Bool
Description
Creates and records a custom event that you can query using NRQL. The event includes a list of attributes, specified as a map. Unlike using setAttribute
, adding attributes to a custom event adds them only to that event; they are not session attributes.
Important considerations and best practices include:
Limit the total number of
eventType
values to approximately five. It is meant to be used for high-level categories, such asGestures.
Do not use
eventType
to name your custom events. Instead, create attributes to name custom events, or use the optionalname
parameter.Use the
name
keyword to display your events in the mobile monitoring UI. To create a useful name, you can combine several attributes. Using thename
parameter has the same effect as adding aname
key in the attributes dictionary.The elements of the attributes object must be of the type NSString or NSNumber.
Parameters
Parameter
Type
Description
$eventType
string
Required. The type of event. Do not use
$eventType
to name your custom events; use a custom attribute or the optionalname
parameter.$eventName
string
Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a
name
parameter.$eventAttributes
dictionary
Optional. A map that includes a list of attributes that further designate subcategories to the
$eventType
. The elements of the attributes object must be of the type NSString or NSNumber.You can create attributes for any event descriptors you think will be useful. To name your custom events, create a
name
attribute or use theeventName
parameter.Important
When setting the key for your custom attributes, be aware that there are default attributes that cannot be overridden.
Return values
Returns
true
if the event is recorded successfully, orfalse
if not.Examples
Objective-C
BOOL eventRecorded = [NewRelic recordCustomEvent:@"eventType"attributes:@{@"attributeName1": @"value1", @"attributeName2": @2}];
BOOL eventRecorded = [NewRelic recordCustomEvent:@"Vehicle" name:@"1908 Ford ModelT"attributes:@{@"make":@"Ford", @"model":@"ModelT", @"year": @1908, @"color": @"black", @"mileage": @250000}];
Swift
let eventRecorded = NewRelic.recordCustomEvent("eventType", attributes: ["attributeName1" : "value1", "attributeName2": 2])
let eventRecorded = NewRelic.recordCustomEvent("Vehicle", name:"1908 Ford ModelT", attributes:["make":"Ford", "model":"ModelT", "year": 1908, "color": "black", "mileage": 250000]);
Syntax
recordCustomEvent(options: { eventType: string; eventName: string; attributes: object; }) => void
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A map that includes a list of attributes that further designate subcategories to the |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelicCapacitorPlugin.recordCustomEvent({ eventType: "mobileClothes", eventName: "pants", attributes:{"pantsColor": "blue","pantssize": 32,"belt": true} });
Syntax
recordCustomEvent(eventType: string, eventName?: string, attributes?: {[key: string]: boolean | number | string}): void;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A map that includes a list of attributes that further designate subcategories to the |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelic.recordCustomEvent("mobileClothes", "pants", {"pantsColor": "blue", "pantssize": 32, "belt": true});
Syntax
RecordCustomEvent(string eventType, string eventName, Dictionary<string, object> attributes): bool;
Description
Creates and records a custom event, for use in NRQL.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A dictionary of key-value pairs that can be used to provide additional information about the custom event. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
CrossNewRelic.Current.RecordCustomEvent("MAUICustomEvent", "MAUICustomEventCategory", new Dictionary<string, object>() { {"BreadNumValue", 12.3 }, {"BreadStrValue", "MAUIBread" }, {"BreadBoolValue", true } });
Syntax
recordCustomEvent(String eventType,{String eventName = "", Map<String, dynamic>? eventAttributes}): void;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A map that includes a list of attributes that further designate subcategories to the |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewrelicMobile.instance.recordCustomEvent("Major",eventName: "User Purchase",eventAttributes: {"item1":"Clothes","price":34.00});
Syntax
recordCustomEvent(eventType: string, eventName?: string, attributes?: {[key: string]: any}): void;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A map that includes a list of attributes that further designate subcategories to the |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelic.recordCustomEvent("mobileClothes", "pants", {"pantsColor": "blue","pantssize": 32,"belt": true});
Syntax
RecordCustomEvent(string name, Dictionary<string, object> attributes): bool;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A dictionary of key-value pairs that can be used to provide additional information about the custom event. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
Dictionary<string, object> dic = new Dictionary<string, object>();dic.Add("Unity Custom Attribute", "Data2");
NewRelicAgent.RecordCustomEvent("Unity Custom Event Example", dic);
Syntax
RecordCustomEvent(string name, Dictionary<string, object> attributes): bool;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A dictionary of key-value pairs that can be used to provide additional information about the custom event. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
Dictionary<string, object> dic = new Dictionary<string, object>();dic.Add("Unity Custom Attribute", "Data2");
NewRelicAgent.RecordCustomEvent("Unity Custom Event Example", dic);
Syntax
recordCustomEvent(FString eventType, TMap <FString, FString> eventAttributes):void
recordCustomEventWithEventType(FString eventType,FString eventName, TMap <FString, FString> eventAttributes):void
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. A map that includes a list of attributes that further designate subcategories to the Note: Not all object types are supported. See setAttribute for details on supported types. ImportantWhen setting the key for your custom attributes, be aware that there are default attributes that cannot be overridden. |
Example
#include "NewRelicBPLibrary.h"
TMap<FString, FString> customEventMap;customEventMap.Add("place", TEXT("Robots"));customEventMap.Add("user", TEXT("user1"));
UNewRelicBPLibrary::recordCustomEvent("Unreal Custom Event Example", customEventMap);
TMap<FString, FString> customEventMap;customEventMap.Add("place", TEXT("Robots"));customEventMap.Add("user", TEXT("user1"));
UNewRelicBPLibrary::recordCustomEvent("Unreal Custom Event Type","Unreal Custom Event Example", customEventMap);
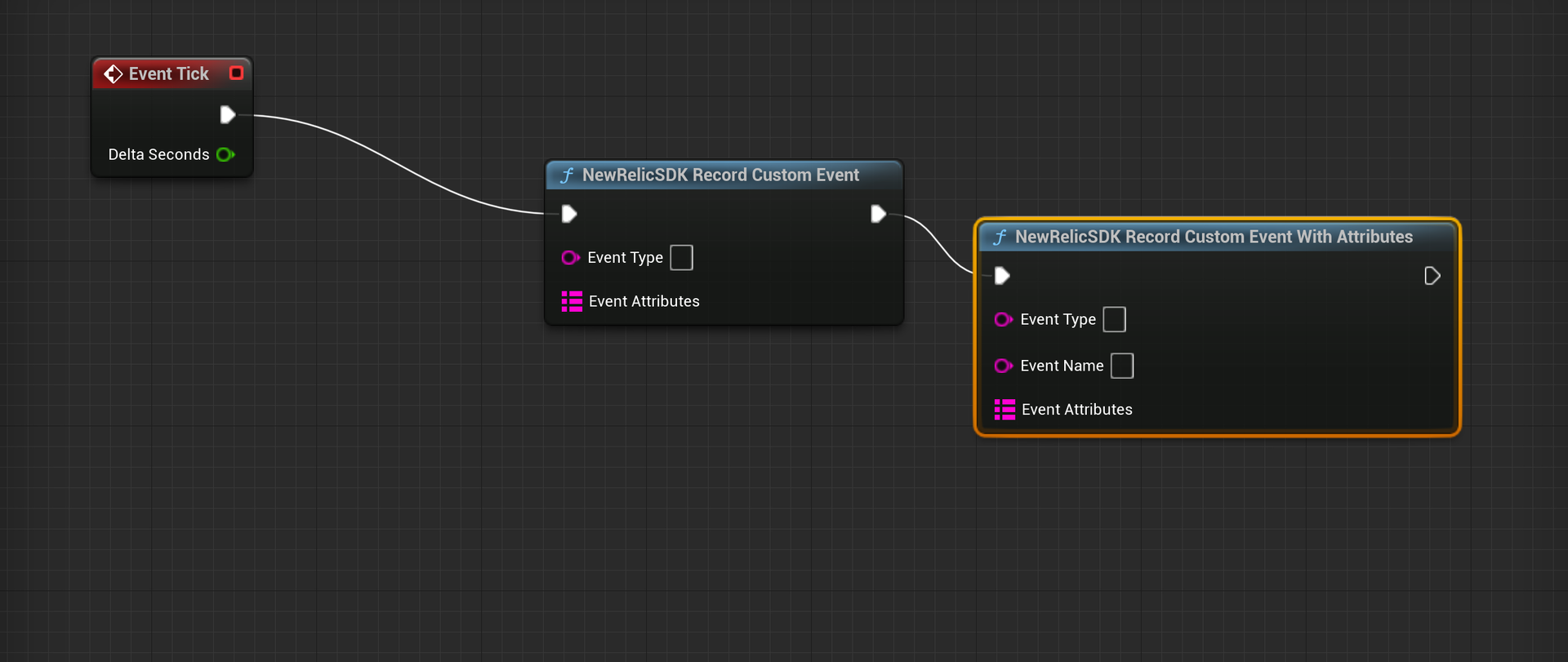
Syntax
RecordCustomEvent(string eventType, string eventName, Dictionary<string, object> attributes): bool;
Description
Creates and records a custom event, for use in NRQL.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The type of event. Do not use |
|
| Optional. Use this parameter to name the event. Using this parameter is equivalent to creating a |
|
| Optional. A dictionary of key-value pairs that can be used to provide additional information about the custom event. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
CrossNewRelicClient.Current.RecordCustomEvent("XamarinCustomEvent", "XamarinCustomEventCategory", new Dictionary<string, object>() { {"BreadNumValue", 12.3 }, {"BreadStrValue", "XamBread" }, {"BreadBoolValue", true } });