Syntax
Java
NewRelic.recordBreadcrumb( string $Name, map<string, object> $eventAttributes )
Kotlin
NewRelic.recordBreadcrumb( breadcrumbName: String?, attributes: MutableMap<String?, Any?>? )
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Examples
Here's an example of a simple breadcrumb event call:
Java
Map attributes = new HashMap();attributes.put("attributeName1", "value1");attributes.put("attributeName1", 2);boolean eventRecorded = NewRelic.recordBreadcrumb("Name", attributes);
Kotlin
val map = mutableMapOf<String,Any>("attributeName1" to "value1", "attributeName1" to 2)val eventRecorded = NewRelic.recordBreadcrumb("Name", map)
Here's an example of a breadcrumb event with real-world values:
Java
Map attributes = new HashMap();attributes.put("button", "sign-in");attributes.put("location", "LaunchFragment");
NewRelic.recordBreadcrumb("user tapped sign in button", attributes);
Kotlin
val attributes = mutableMapOf<String,Any>("button" to "sign-in", "location" to "LaunchFragment")NewRelic.recordBreadcrumb("user tapped sign in button", attributes)
Syntax
Objective-c
recordBreadcrumb:(NSString* __nonnull)name attributes:(NSDictionary* __nullable)attributes;
Swift
NewRelic.recordBreadcrumb(string $Name, map $eventAttributes)
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; create them for app activity that you think will help you troubleshoot crashes.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Using this call has the same result as using the recordCustomEvent
call with MobileBreadcrumb
as the event type. For context on how to use this API, read about sending custom attributes and events:
Parameters
Parameter
Type
Description
$Name
string
Required. The name you want to give to the breadcrumb event.
$eventAttributes
dictionary
Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful.
Return values
Returns
true
if the event is recorded successfully, orfalse
if not.Examples
Objective-C
[NewRelic recordBreadcrumb:@"user 11-3435 tapped sign-in button on LaunchViewController"attributes:@{ @"button" : @"sign-in", @"action" : @"tapped", @"userId" : @"11-3435", @"location" : @"LaunchViewController"}];
Swift
let eventRecorded = NewRelic.recordBreadcrumb("user 11-3435 tapped sign-in from LaunchViewController", attributes:["button" : "sign-in", "userId" : "11-3435", "action" : "tapped", "location" : "LaunchViewController"])
Syntax
recordBreadcrumb(options: { name: string; eventAttributes: object; }) => void
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelicCapacitorPlugin.recordBreadcrumb({ name: "shoe", eventAttributes: {"shoeColor": "blue","shoesize": 9,"shoeLaces": true} });
Syntax
recordBreadcrumb(eventName: string, attributes?: {[key: string]: boolean | number | string}): void;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
| String | Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelic.recordBreadcrumb("shoe", {"shoeColor": "blue","shoesize": 9,"shoeLaces": true});
Syntax
RecordBreadcrumb(string name, Dictionary<string, object> attributes): bool;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to any custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
CrossNewRelic.Current.RecordBreadcrumb("MAUIExampleBreadcrumb", new Dictionary<string, object>() { {"BreadNumValue", 12.3 }, {"BreadStrValue", "MAUIBread" }, {"BreadBoolValue", true } });
Syntax
recordBreadcrumb(String name,{Map<String, dynamic>? eventAttributes}): void;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to whatever custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewrelicMobile.instance.recordBreadcrumb("Button Got Pressed on Screen 3");
Syntax
recordBreadcrumb(name: string, attributes?: {[key: string]: any}): void;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to whatever custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
NewRelic.recordBreadcrumb("shoe", {"shoeColor": "blue","shoesize": 9,"shoeLaces": true});
Syntax
RecordBreadcrumb(string name, Dictionary<string, object> attributes): bool;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to whatever custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
Dictionary<string, object> dic = new Dictionary<string, object>();dic.Add("Unity Attribute", "Data1");
NewRelicAgent.RecordBreadCrumb("Unity BreadCrumb Example", dic);
Syntax
recordBreadCrumb(FString breadcrumbName);
recordBreadCrumbWithAttributes(FString breadcrumbName, TMap <FString, FString> customData):void
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to whatever custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Example
#include "NewRelicBPLibrary.h"
UNewRelicBPLibrary::.recordBreadCrumb("Unreal Breadcrumb Without Attribute");
TMap<FString, FString> breadCrumbMap;breadCrumbMap.Add("place", TEXT("Robots"));breadCrumbMap.Add("user", TEXT("Nisarg"));
UNewRelicBPLibrary::recordBreadCrumbWithAttributes("Unreal Breadcrumb",breadCrumbMap);
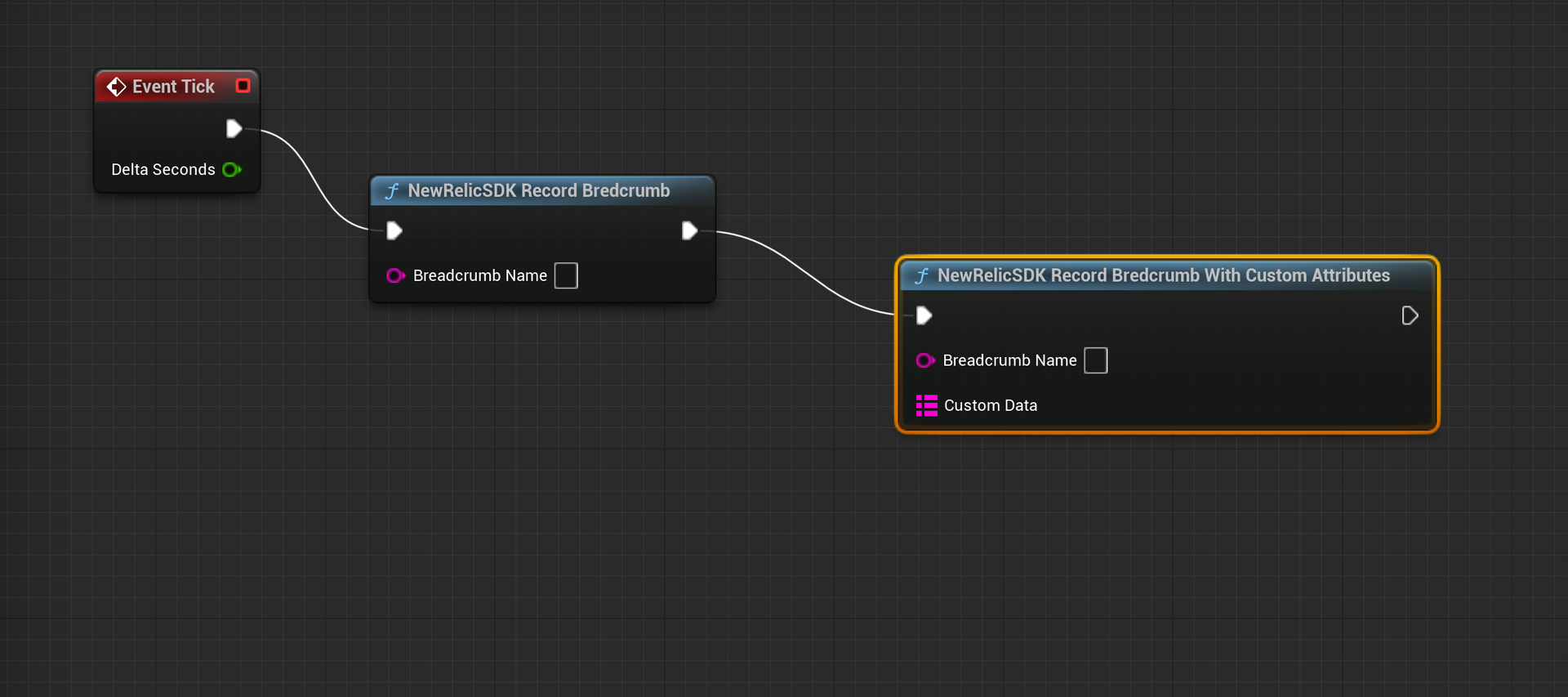
Syntax
RecordBreadcrumb(string name, Dictionary<string, object> attributes): bool;
Description
This call creates and records a MobileBreadcrumb
event, which can be queried with NRQL and in the crash event trail. Mobile breadcrumbs are useful for crash analysis; they should be created for app activity that may be helpful for troubleshooting crashes.
In addition to whatever custom attributes you choose, the event will also have associated session attributes. Unlike with using setAttribute
, adding attributes to a breadcrumb event adds them only to that event; they are not session attributes.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The name you want to give to the breadcrumb event. |
|
| Optional. A map that includes a list of attributes of the breadcrumb event. Create attributes for any event descriptors you think will be useful. |
Return values
Returns true
if the event is recorded successfully, or false
if not.
Example
CrossNewRelicClient.Current.RecordBreadcrumb("XamarinExampleBreadcrumb", new Dictionary<string, object>() { {"BreadNumValue", 12.3 }, {"BreadStrValue", "XamBread" }, {"BreadBoolValue", true } });