Syntax
Java
NewRelic.endInteraction(string $interactionID)
Kotlin
NewRelic. endInteraction(id : String!)
Description
New Relic ends all interactions automatically, but you can use endInteraction()
to end a custom interaction early. The string ID is returned when you use the startInteraction()
call.
This call has no effect if the interaction has already ended.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
Here's an example of ending a custom interaction RefreshContacts
:
Java
public class MainActivity extends Activity { ... @Override public boolean onOptionsItemSelected(MenuItem item) { switch (item.getItemId()) { case R.id.action_refresh: String interactionId = NewRelic.startInteraction("RefreshContacts"); ... return true; default: NewRelic.endInteraction(interactionId); return super.onOptionsItemSelected(item); } } ...}
Syntax
Objective-c
+ (void) stopCurrentInteraction:(NSString*)interactionIdentifier;
Swift
NewRelic.stopInteraction(string: "myInteractionName")
Description
This method will stop the interaction trace associated with the interactionIdentifier
(which is returned by the startInteractionWithName:
method). It's not necessary to call this method to complete an interaction trace (an interaction trace will intelligently complete on its own). However, use this method if you want a more discrete interaction period.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Examples
Objective-C
NSString *identifier = [NewRelic startInteractionWithName: @"myInteractionName"];[NewRelic stopCurrentInteraction: identifier];
Swift
let identifier = NewRelic.startInteraction(withName: "myInteractionName")NewRelic.stopCurrentInteraction(identifier)
Syntax
endInteraction(options: { interactionId: string; }) => void
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
const badApiLoad = async () => { const id = await NewRelicCapacitorPlugin.startInteraction({ value: 'StartLoadBadApiCall' }); console.log(id); const url = 'https://fakewebsite.com/moviessssssssss.json'; fetch(url) .then((response) => response.json()) .then((responseJson) => { console.log(responseJson); NewRelicCapacitorPlugin.endInteraction({ interactionId: id.value }); }) .catch((error) => { NewRelicCapacitorPlugin.endInteraction({ interactionId: id.value }); console.error(error); });};
Syntax
endInteraction(id: InteractionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
const badApiLoad = async () => { const interactionId = await NewRelic.startInteraction('StartLoadBadApiCall'); console.log(interactionId); const url = 'https://cordova.apache.org/moviessssssssss.json'; fetch(url) .then((response) => response.json()) .then((responseJson) => { console.log(responseJson); NewRelic.endInteraction(interactionId); }) .catch((error) => { NewRelic.endInteraction(interactionId); console.error(error); });
Syntax
EndInteraction(string interactionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
HttpClient myClient = new HttpClient(CrossNewRelic.Current.GetHttpMessageHandler());string interactionId = CrossNewRelic.Current.StartInteraction("Getting data from service");var response = await myClient.GetAsync(new Uri("https://jsonplaceholder.typicode.com/todos/1"));
if (response.IsSuccessStatusCode){ var content = await response.Content.ReadAsStringAsync();} else{ Console.WriteLine("Unsuccessful response code");}
CrossNewRelic.Current.EndInteraction(interactionId);
Syntax
endInteraction(String interactionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
var id = await NewrelicMobile.instance.startInteraction("Getting Data from Service");
try { var dio = Dio(); var response = await dio.get( 'https://reqres.in/api/users?delay=15'); print(response); NewrelicMobile.instance.endInteraction(id); Timeline.finishSync();} catch (e) { print(e);}
Syntax
endInteraction(id: InteractionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
const badApiLoad = async () => { const interactionId = await NewRelic.startInteraction('StartLoadBadApiCall'); console.log(interactionId); const url = 'https://facebook.github.io/react-native/moviessssssssss.json'; fetch(url) .then((response) => response.json()) .then((responseJson) => { console.log(responseJson); NewRelic.endInteraction(interactionId); }) .catch((error) => { NewRelic.endInteraction(interactionId); console.error(error); });;};
Syntax
StopCurrentInteraction(string interactionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
string interActionId = NewRelicAgent.StartInteractionWithName("Unity InterAction Example");
for(int i =0; i < 4;i++){ Thread.Sleep(1000);}
NewRelicAgent.StopCurrentInteraction(interActionId);
Syntax
endInterAction(FString interActionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
#include "NewRelicBPLibrary.h"
FString id = UNewRelicBPLibrary::startInterAction("test Unreal InterAction");
FPlatformProcess::Sleep(6.0);
UNewRelicBPLibrary::endInterAction(id);
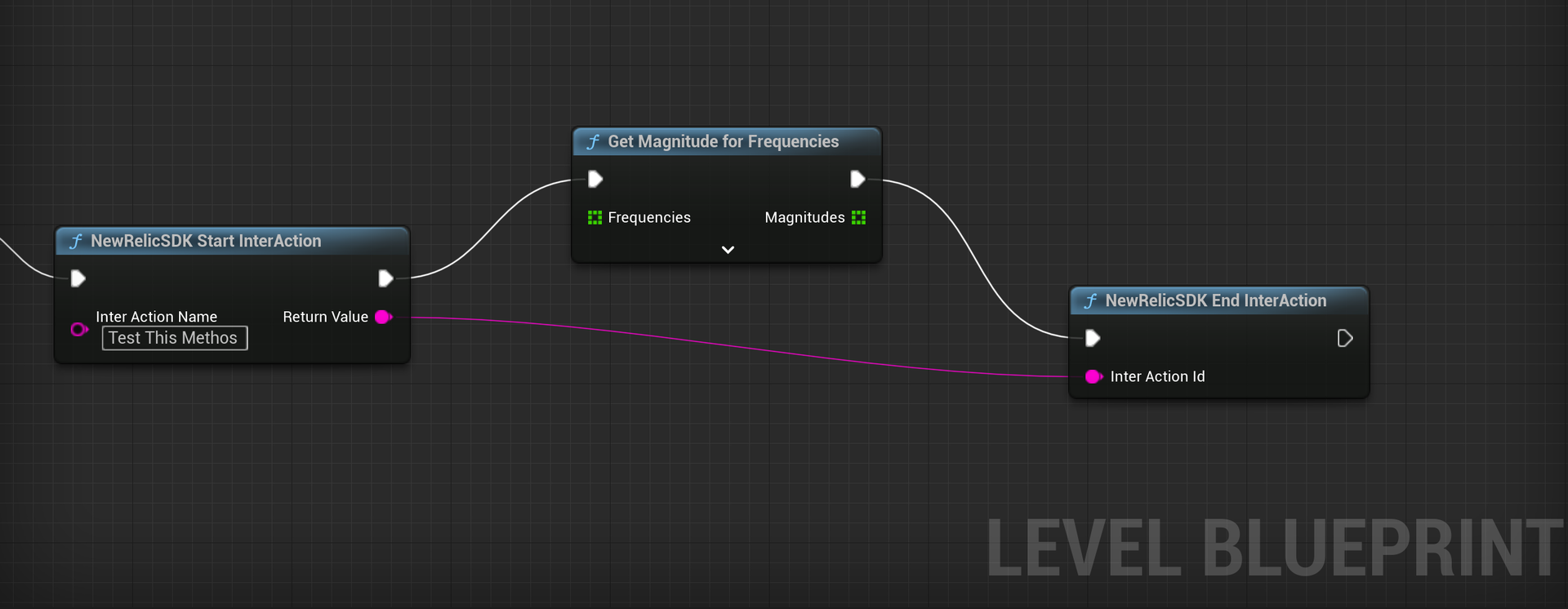
Syntax
EndInteraction(string interactionId): void;
Description
This uses the string ID for the interaction you want to end. This string is returned when you use startInteraction()
.
Parameters
Parameter | Type | Description |
---|---|---|
|
| Required. The value returned by |
Example
HttpClient myClient = new HttpClient(CrossNewRelicClient.Current.GetHttpMessageHandler());
string interactionId = CrossNewRelicClient.Current.StartInteraction("Getting data from service");var response = await myClient.GetAsync(new Uri("https://jsonplaceholder.typicode.com/todos/1"));
if (response.IsSuccessStatusCode){ var content = await response.Content.ReadAsStringAsync();}else{ Console.WriteLine("Unsuccessful response code");}
CrossNewRelicClient.Current.EndInteraction(interactionId);